JavaScript HTML DOM Navigation
With the HTML DOM, you can navigate the node tree using node relationships.
DOM Nodes
According to the W3C HTML DOM standard, everything in an HTML document is a node:
- The entire document is a document node
- Every HTML element is an element node
- The text inside HTML elements are text nodes
- Every HTML attribute is an attribute node
- All comments are comment nodes
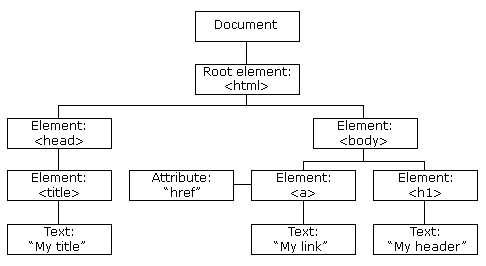
With the HTML DOM, all nodes in the node tree can be accessed by JavaScript.
New nodes can be created, and all nodes can be modified or deleted.
Node Relationships
The nodes in the node tree have a hierarchical relationship to each other.
The terms parent, child, and sibling are used to describe the relationships.
- In a node tree, the top node is called the root (or root node)
- Every node has exactly one parent, except the root (which has no parent)
- A node can have a number of children
- Siblings (brothers or sisters) are nodes with the same parent
<html>
<head>
<title>DOM Tutorial</title>
</head>
<body>
<h1>DOM Lesson one</h1>
<p>Hello world!</p>
</body>
</html>
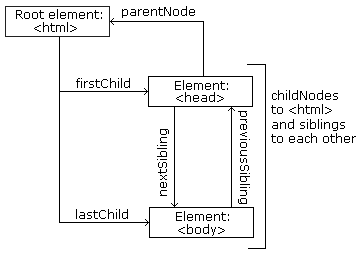
From the HTML above you can read:
- <html> is the root node
- <html> has no parents
- <html> is the parent of <head> and <body>
- <head> is the first child of <html>
- <body> is the last child of <html>
and:
- <head> has one child: <title>
- <title> has one child (a text node): "DOM Tutorial"
- <body> has two children: <h1> and <p>
- <h1> has one child: "DOM Lesson one"
- <p> has one child: "Hello world!"
- <h1> and <p> are siblings
Navigating Between Nodes
You can use the following node properties to navigate between nodes with JavaScript:
- parentNode
- childNodes[nodenumber]
- firstChild
- lastChild
- nextSibling
- previousSibling
Warning !
A common error in DOM processing is to expect an element node to contain text.
In this example: <title>DOM Tutorial</title>, the element node <title> does not contain text. It contains a text node with the value "DOM Tutorial".
The value of the text node can be accessed by the node's innerHTML property, or the nodeValue.
Child Nodes and Node Values
In addition to the innerHTML property, you can also use the childNodes and nodeValue properties to get the content of an element.
The following example collects the node value of an <h1> element and copies it into a <p> element:
Example
<html>
<body>
<h1 id="intro">My First Page</h1>
<p id="demo">Hello!</p>
<script>
var myText =
document.getElementById("intro").childNodes[0].nodeValue;
document.getElementById("demo").innerHTML =
myText;
</script>
</body>
</html>
Try it Yourself »
In the example above, getElementById is a method, while childNodes and nodeValue are properties.
In this tutorial we use the innerHTML property. However, learning the method above is useful for understanding the tree structure and the navigation of the DOM.
Using the firstChild property is the same as using childNodes[0]:
Example
<html>
<body>
<h1 id="intro">My First Page</h1>
<p id="demo">Hello World!</p>
<script>
myText = document.getElementById("intro").firstChild.nodeValue;
document.getElementById("demo").innerHTML = myText;
</script>
</body>
</html>
Try it Yourself »
DOM Root Nodes
There are two special properties that allow access to the full document:
- document.body - The body of the document
- document.documentElement - The full document
Example
<html>
<body>
<p>Hello World!</p>
<div>
<p>The DOM is very useful!</p>
<p>This example demonstrates the <b>document.body</b> property.</p>
</div>
<script>
alert(document.body.innerHTML);
</script>
</body>
</html>
Try it Yourself »
Example
<html>
<body>
<p>Hello World!</p>
<div>
<p>The DOM is very useful!</p>
<p>This example demonstrates the <b>document.documentElement</b> property.</p>
</div>
<script>
alert(document.documentElement.innerHTML);
</script>
</body>
</html>
Try it Yourself »
The nodeName Property
The nodeName property specifies the name of a node.
- nodeName is read-only
- nodeName of an element node is the same as the tag name
- nodeName of an attribute node is the attribute name
- nodeName of a text node is always #text
- nodeName of the document node is always #document
Note: nodeName always contains the uppercase tag name of an HTML element.
The nodeValue Property
The nodeValue property specifies the value of a node.
- nodeValue for element nodes is undefined
- nodeValue for text nodes is the text itself
- nodeValue for attribute nodes is the attribute value
The nodeType Property
The nodeType property returns the type of node. nodeType is read only.
The most important node types are:
Element type | NodeType |
---|---|
Element | 1 |
Attribute | 2 |
Text | 3 |
Comment | 8 |
Document | 9 |